odrivetool
The odrivetool
is the accompanying PC program for the ODrive. Its main purpose is to provide an interactive shell to control the device manually, as well as some supporting functions like firmware update.
Installation
Install Python 3. We recommend the Anaconda distribution because it packs a lot of useful scientific tools, however you can also install the standalone python.
Anaconda: Download the installer from here. Execute the downloaded file and follow the instructions.
Standalone Python: Download the installer for 3.8.6 from here. Execute the downloaded file and follow the instructions. As of Oct 2020, Matplotlib (required by odrivetool) had not been updated to work with 3.9, so please use 3.8.6.
If you have Python 2 installed alongside Python 3, replace
pip
byC:\Users\YOUR_USERNAME\AppData\Local\Programs\Python\Python36-32\Scripts\pip
. If you have trouble with this step then refer to this walkthrough.
Launch the command prompt.
Anaconda: In the start menu, type
Anaconda Prompt
EnterStandalone Python: In the start menu, type
cmd
Enter
The remaining instructions are commands to be typed into the command prompt.
Optional: Install matplotlib (only needed if you’re going to use the Liveplotter).
pip install matplotlib
Install
odrivetool
.pip install --upgrade odrive
We are going to run the following commands for installation in Terminal.
Install homebrew. You can skip this step if
brew doctor
already returns something likeYour system is ready to brew.
./usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)" echo 'export PATH="/opt/homebrew/bin:$PATH"' >> ~/.zshrc echo 'export DYLD_LIBRARY_PATH="/opt/homebrew/lib:$DYLD_LIBRARY_PATH"' >> ~/.zshrc source ~/.zshrc
Install Python 3. You can skip this step if
python3 --version
already returns something likePython 3.6.0
or newer.brew install python3
Optional: Install libusb (only needed for Device Firmware Updates).
brew install libusb
Optional: Install matplotlib (only needed if you’re going to use the Liveplotter).
pip3 install matplotlib
Install
odrivetool
.pip3 install --upgrade pip pip3 install --upgrade odrive echo 'export PATH="'$(python3 -c "import sysconfig; print(sysconfig.get_path('scripts', 'posix_user'))")':$PATH"' >> ~/.zshrc source ~/.zshrc
Troubleshooting
Permission Errors: Just run the previous command in sudo
sudo pip3 install --upgrade odrive
Dependency Errors: If the installer doesn’t complete, and you get a dependency error (Ex. “No module…” or “module_name not found”)
sudo pip3 install module_name
Try step 5 again.
Other Install Errors: If the installer fails at installing dependencies, try
sudo pip3 install odrive --no-deps
If you do this, brace yourself for runtime errors when you run
odrivetool
(the basic functionality should work though).
Install Python 3. You can skip this step if
python3 --version
already returns something likePython 3.6.0
or newer. The installation command depends on your Linux distribution. For example on Ubuntu:sudo apt install python3 python3-pip
If this doesn’t work, see the official download page.
Optional: Install matplotlib (only needed if you’re going to use the Liveplotter).
pip3 install matplotlib
Install
odrivetool
.pip3 install --upgrade odrive
Set up USB device permissions.
sudo bash -c "curl https://cdn.odriverobotics.com/files/odrive-udev-rules.rules > /etc/udev/rules.d/91-odrive.rules && udevadm control --reload-rules && udevadm trigger"
Ubuntu, Raspbian: If you can’t invoke
odrivetool
at this point, try adding~/.local/bin
to your$PATH
(see related bug). This is done for example by runningnano ~/.bashrc
, scrolling to the bottom, pastingexport PATH=$PATH:~/.local/bin
, and then saving and closing, and close and reopen the terminal window.
Start odrivetool
To launch the main interactive ODrive tool, type odrivetool
Enter. Connect your ODrive and wait for the tool to find it. If it doesn’t connect after a few seconds refer to the troubleshooting page. Now you can, for instance type odrv0.vbus_voltage
Enter to inspect the boards main supply voltage.
It should look something like this:
ODrive control utility v0.5.1
Please connect your ODrive.
Type help() for help.
Connected to ODrive 306A396A3235 as odrv0
In [1]: odrv0.vbus_voltage
Out[1]: 11.97055721282959
The tool you’re looking at is a fully capable Python command prompt, so you can type any valid python code.
Hint
If any of the following steps fail, print the errors by running dump_errors(odrv0)
in odrivetool
. You can clear errors by running odrv0.clear_errors()
.
Type odrivetool --help
to see what features are available.
Multiple ODrives
By default, odrivetool
will connect to any ODrive it finds.
If this is not what you want, you can select a specific ODrive.
To find the serial number of your ODrive, run odrivetool
, connect exactly one ODrive and power it up.
You should see this:
Connected to ODrive 306A396A3235 as odrv0
In [1]:
306A396A3235
is the serial number of this particular ODrive.
If you want odrivetool
to ignore all other devices you would close it and then run:
odrivetool --serial-number 306A396A3235
My ODrive is stuck in DFU mode, can I still find the serial number?
Yes, the serial number is part of the USB descriptors. In Linux you can find it by running:
(sudo lsusb -d 1209:0d32 -v; sudo lsusb -d 0483:df11 -v) 2>/dev/null | grep iSerial
This should output something like:
iSerial 3 385F324D3037 iSerial 3 306A396A3235
Here, two ODrives are connected.
Commands and Objects
The ODrive Tool console provides aliases and wrappers for various entities in the odrive
Python package. These are optimized for interactive use. When you write your own Python script that does import odrive
, these wrappers are not available.
- shell.dump_errors(odrv: ODrive)
Alias for
odrive.utils.dump_errors()
- shell.start_liveplotter(*args)
Alias for
odrive.utils.start_liveplotter()
- shell.status(config: odrive.config.MachineConfig)
Wrapper for
config.show_status(odrives)
- shell.apply(config: odrive.config.MachineConfig)
Wrapper for
config.apply(odrives)
- shell.calibrate(config: odrive.config.MachineConfig)
Wrapper for
config.calibrate(odrives)
Liveplotter
Liveplotter is used for the graphical plotting of ODrive parameters (i.e. position) in real time.
Note
This is an experimental feature. Please use the Web GUI for liveplotting instead.
Example 1
In this example we plot the position of two motors. They are both running in AxisState.CLOSED_LOOP_CONTROL
while they are being forced off position by hand.
start_liveplotter(lambda: [
odrv0.axis0.pos_estimate,
odrv1.axis0.pos_estimate,
])
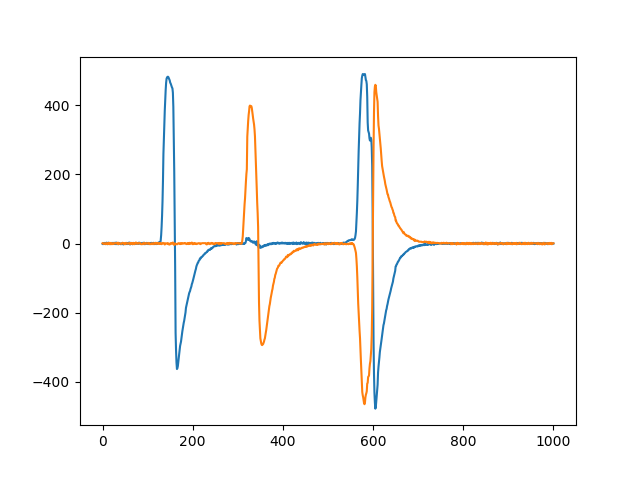
Example 2
In this example we plot the approximate motor torque [Nm] and the velocity [RPM] of axis0.
start_liveplotter(lambda: [
odrv0.axis0.pos_vel_mapper.vel * 60, # turns/s to rpm
odrv0.axis0.motor.foc.Iq_setpoint * odrv0.axis0.config.motor.torque_constant, # Torque [Nm]
])
The motor is forced off axis by hand and held there. In response the motor controller increases the torque (orange line) to counteract this disturbance up to a peak of 500 N.cm at which point the motor current limit is reached. When the motor is released it returns to its commanded position very quickly as can be seen by the spike in the motor velocity (blue line).
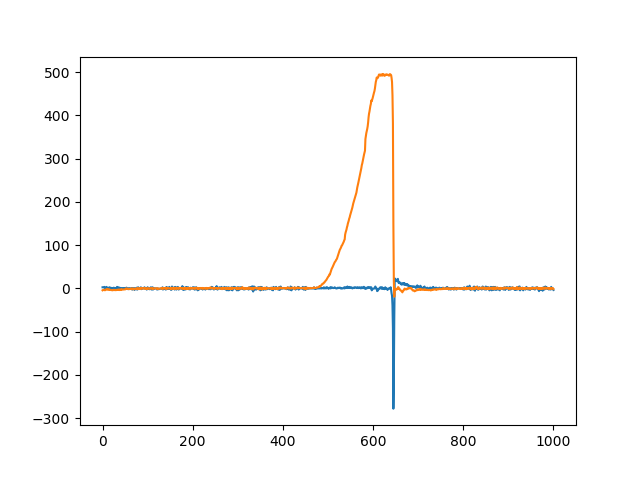
Liveplotter Torque Velocity Plot
Liveplotter from Interactive odrivetool
Instance
You can also run start_liveplotter(...)
directly from the interactive odrivetool prompt.
This is useful if you want to issue commands or otherwise keep interacting with the odrive while plotting.
For example, you can type the following directly into the interactive prompt:
start_liveplotter(lambda: [odrv0.axis0.pos_estimate])
Just like the examples above, you can list several parameters to plot separated by comma in the square brackets. In general, you can plot any variable that you are able to read like normal in odrivetool.
Configuration Backup
You can use odrivetool
to back up and restore device configurations or transfer the configuration of one ODrive to another one.
To save the configuration to a file on the PC, run
odrivetool backup-config my_config.jsonTo restore the configuration form such a file, run
odrivetool restore-config my_config.json
Note
The encoder offset calibration is not restored because this would be dangerous if you transfer the calibration values of one axis to another axis.
Note
It is not recommended to transfer the configuration between different firmware versions because variables can get renamed
or removed (in which case odrivetool
will show warnings) or defaults changed between firmware versions.
After firmware updates it is instead recommended to check the CHANGELOG and manually transfer the settings.